在本文中,您将开始创建根据 MVVM 模式构建的示例应用程序,并学习如何使用 Scaffolding Wizard。
1. 创建一个新的WinForms应用程序。 要创建项目的数据模型,请添加一个新的模型文件夹并使用下面的代码片段创建三个类——帐户、交易和类别,这些类使用数据注释属性并要求将 System.ComponentModel.DataAnnotations 库包含在您的项目中。
账户数据模型
C#
using System.ComponentModel.DataAnnotations; namespace MVVMExpenses.DataModels { public class Account { [Key, Display(AutoGenerateField = false)] public long ID { get; set; } [Required, StringLength(30, MinimumLength = 4)] [Display(Name = "ACCOUNT")] public string Name { get; set; } [DataType(DataType.Currency)] [Display(Name = "AMOUNT")] public decimal Amount { get; set; } public override string ToString() { return Name + " (" + Amount.ToString("C") + ")"; } public virtual ICollection<Transaction> Transactions { get; set; } } }
VB.NET
Imports System.ComponentModel.DataAnnotations Namespace MVVMExpenses.DataModels Public Class Account <Key, Display(AutoGenerateField := False)> Public Property ID() As Long <Required, StringLength(30, MinimumLength := 4), Display(Name := "ACCOUNT")> Public Property Name() As String <DataType(DataType.Currency), Display(Name := "AMOUNT")> Public Property Amount() As Decimal Public Overrides Function ToString() As String Return Name & " (" & Amount.ToString("C") & ")" End Function Public Overridable Property Transactions() As ICollection(Of Transaction) End Class End Namespace
类别数据模型
C#
using System.ComponentModel.DataAnnotations; namespace MVVMExpenses.DataModels { public class Category { [Key, Display(AutoGenerateField = false)] public long ID { get; set; } [Required, StringLength(30, MinimumLength = 5)] [Display(Name = "CATEGORY")] public string Name { get; set; } [EnumDataType(typeof(TransactionType))] [Display(Name = "TRANSACTION TYPE")] public TransactionType Type { get; set; } public override string ToString() { return Name + " (" + Type.ToString() + ")"; } public virtual ICollection<Transaction> Transactions { get; set; } } }
VB.NET
Namespace MVVMExpenses.DataModels Public Class Category <Key, Display(AutoGenerateField := False)> Public Property ID() As Long <Required, StringLength(30, MinimumLength := 5), Display(Name := "CATEGORY")> Public Property Name() As String <EnumDataType(GetType(TransactionType)), Display(Name := "TRANSACTION TYPE")> Public Property Type() As TransactionType Public Overrides Function ToString() As String Return Name & " (" & Type.ToString() & ")" End Function Public Overridable Property Transactions() As ICollection(Of Transaction) End Class End Namespace
交易数据模型
C#
using System.ComponentModel.DataAnnotations; namespace MVVMExpenses.DataModels { public enum TransactionType { Expense, Income } public class Transaction { [Key, Display(AutoGenerateField = false)] public long ID { get; set; } [Display(AutoGenerateField = false)] public long AccountID { get; set; } [Display(Name = "ACCOUNT")] public virtual Account Account { get; set; } [Display(AutoGenerateField = false)] public long CategoryID { get; set; } [Display(Name = "CATEGORY")] public virtual Category Category { get; set; } [DataType(DataType.Date)] [Display(Name = "DATE")] public DateTime Date { get; set; } [DataType(DataType.Currency)] [Display(Name = "AMOUNT")] public decimal Amount { get; set; } [DataType(DataType.MultilineText)] [Display(Name = "COMMENT")] public string Comment { get; set; } } }
VB.NET
Namespace MVVMExpenses.DataModels Public Enum TransactionType Expense Income End Enum Public Class Transaction <Key, Display(AutoGenerateField := False)> Public Property ID() As Long <Display(AutoGenerateField := False)> Public Property AccountID() As Long <Display(Name := "ACCOUNT")> Public Overridable Property Account() As Account <Display(AutoGenerateField := False)> Public Property CategoryID() As Long <Display(Name := "CATEGORY")> Public Overridable Property Category() As Category <DataType(DataType.Date), Display(Name := "DATE")> Public Property [Date]() As Date <DataType(DataType.Currency), Display(Name := "AMOUNT")> Public Property Amount() As Decimal <DataType(DataType.MultilineText), Display(Name := "COMMENT")> Public Property Comment() As String End Class End Namespace
2. 跳转到Visual Studio 的 Project 菜单并选择 Manage NuGet Packages...菜单项,在在线存储中搜索 Entity Framework 6 package,然后单击 ‘Install’,请注意如果未安装此 NuGet 包,您将无法在此示例应用程序中使用实体模型。
3. 添加以下 DbContext 类后代,它将用作 DevExpress Scaffolding Wizard 的上下文。
C#
using System.Data.Entity; using MVVMExpenses.DataModels; namespace MVVMExpenses.DataBase { public class MyDbContext : System.Data.Entity.DbContext { static MyDbContext() { System.Data.Entity.Database.SetInitializer<MyDbContext>(null); } public DbSet<Account> Accounts { get; set; } public DbSet<Category> Categories { get; set; } public DbSet<Transaction> Transactions { get; set; } } }
VB.NET
Imports System.Data.Entity Imports MVVMExpenses.DataModels Namespace MVVMExpenses.DataBase Public Class MyDbContext Inherits System.Data.Entity.DbContext Shared Sub New() System.Data.Entity.Database.SetInitializer(Of MyDbContext)(Nothing) End Sub Public Property Accounts() As DbSet(Of Account) Public Property Categories() As DbSet(Of Category) Public Property Transactions() As DbSet(Of Transaction) End Class End Namespace
4. 在继续下一步之前,构建您的项目并确保它编译时没有错误。 然后,在 Visual Studio 解决方案资源管理器中右键单击您的项目并选择 Add DevExpress Item - Data Model Scaffolding...(参见下图)。 这将启动 Scaffolding Wizard,它将生成完整的应用程序层次结构,包括来自数据上下文的 MVVM-ready DataModel。
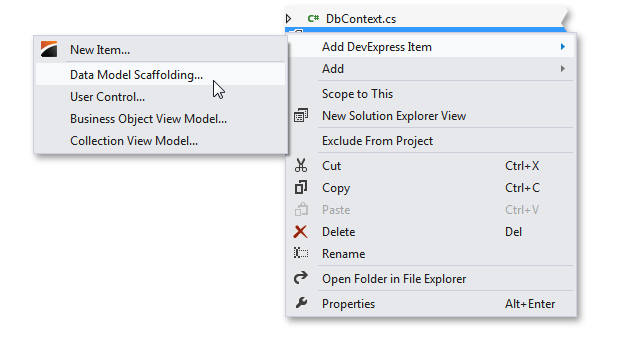
注意:如果缺少此菜单项,请右键单击您的项目并选择默认的 Visual Studio Add - New Item... 选项。 在对话框中,选择 DevExpress Template Gallery 并手动启动 Scaffolding Wizard - 选择 Data Model Scaffolding 选项卡(WinForms Data Models 组)并单击右下角的 Run Wizard 按钮。
在 Wizard 对话框中,选择在第二步中创建的上下文,然后单击 Next。 检查所有必需的表和视图,然后单击Finish来启动模型生成。
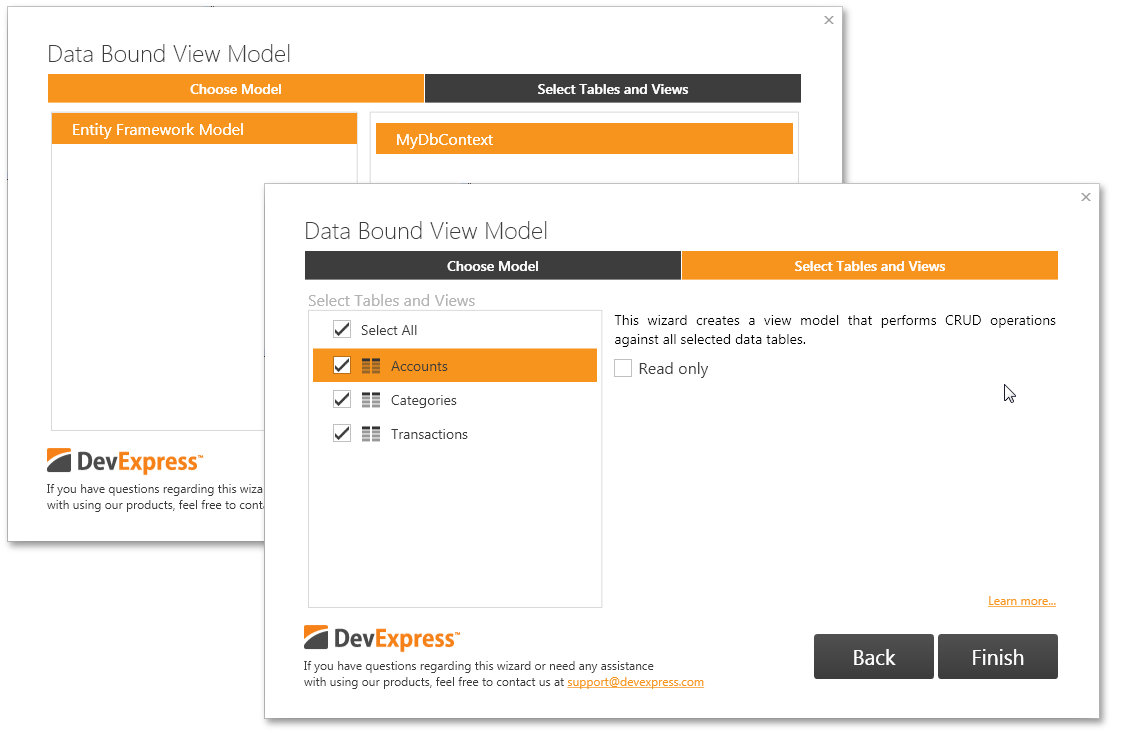
5. 结果,您将看到许多新文件、文件夹和类添加到您的应用程序中。
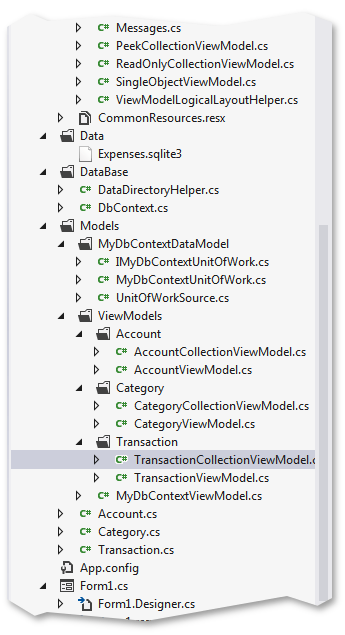
目前,只有向导生成的两个文件夹值得一提 - 包含您的 CRUD 数据模型的 DataModel 文件夹和包含 DbContext 中每个表的 MVVM ViewModel 的 ViewModels 文件夹 - Account、Category 和 Transaction。 在接下来的课程中,您将学习如何为这些 ViewModel 创建 View。
DevExpress WinForm拥有180+组件和UI库,能为Windows Forms平台创建具有影响力的业务解决方案。DevExpress WinForms能完美构建流畅、美观且易于使用的应用程序,无论是Office风格的界面,还是分析处理大批量的业务数据,它都能轻松胜任!
更多产品正版授权详情及优惠,欢迎咨询在线客服>>
DevExpress技术交流群5:742234706 欢迎一起进群讨论
更多DevExpress线上公开课、中文教程资讯请上中文网获取

欢迎任何形式的转载,但请务必注明出处,尊重他人劳动成果
转载请注明:文章转载自:DevExpress控件中文网 [https://www.devexpresscn.com/]
本文地址:https://www.devexpresscn.com/post/2930.html