点击获取DevExpress Winformv21.2正式版
使用缓存提高界面响应速度
前面介绍了通过扩展函数来进一步扩展我们绑定字典类别的方式了,如下扩展函数所示。
/// <summary> /// 绑定下拉列表控件为指定的数据字典列表 /// </summary> /// <param name="combo">下拉列表控件</param> /// <param name="dictTypeName">数据字典类型名称</param> /// <param name="defaultValue">控件默认值</param> public static void BindDictItems(this ComboBoxEdit combo, string dictTypeName, string defaultValue) { Dictionary<string, string> dict = BLLFactory<DictData>.Instance.GetDictByDictType(dictTypeName); List<CListItem> itemList = new List<CListItem>(); foreach (string key in dict.Keys) { itemList.Add(new CListItem(key, dict[key])); } BindDictItems(combo, itemList, defaultValue); }
如果是基于服务接口的方式(通过Web API或者WCF方式)获取字典列表,那么BLLFactory<T>的方式就修改为CallerFactory<T>的方式获取数据了,如下扩展函数所示。
/// <summary> /// 绑定下拉列表控件为指定的数据字典列表 /// </summary> /// <param name="control">下拉列表控件</param> /// <param name="dictTypeName">数据字典类型名称</param> /// <param name="defaultValue">控件默认值</param> /// <param name="emptyFlag">是否添加空行</param> public static void BindDictItems(this ComboBoxEdit control, string dictTypeName, string defaultValue, bool emptyFlag = true) { Dictionary<string, string> dict = CallerFactory<IDictDataService>.Instance.GetDictByDictType(dictTypeName); List<CListItem> itemList = new List<CListItem>(); foreach (string key in dict.Keys) { itemList.Add(new CListItem(key, dict[key])); } control.BindDictItems(itemList, defaultValue, emptyFlag); }
也就是通过服务接口工厂方法调用:
CallerFactory<IDictDataService>.Instance.GetDictByDictType(dictTypeName);
而获取数据字典列表的内容,这个可以配置为Web API访问方式、WCF访问方式,底层就是调用客户端封装的代理方法获取就是了。例如对于Web API调用来说就是通过客户端直接访问Web API服务接口获取数据的,实现代码如下所示。
/// <summary> /// 根据字典类型名称获取所有该类型的字典列表集合(Key为名称,Value为值) /// </summary> /// <param name="dictTypeName">字典类型名称</param> /// <returns></returns> public Dictionary<string, string> GetDictByDictType(string dictTypeName) { var action = System.Reflection.MethodBase.GetCurrentMethod().Name; string url = GetTokenUrl(action) + string.Format("&dictTypeName={0}", dictTypeName.UrlEncode()); Dictionary<string, string> result = JsonHelper<Dictionary<string, string>>.ConvertJson(url); return result; }
由于字典数据是相对比较固定的,一般时效不是那么及时都没问题,由于这部分数据是通过网络的方式获取的,反复的调用获取是会耗费一定的时间。
为了提高用户响应速度,我们可以把它放到客户端的缓存里面(非服务器缓存),设置一定的失效时间,在失效时间内,我们数据不再反复的从网络接口获取,而是直接通过缓存里面提取,速度非常快,同时也提高了界面响应速度。
但是为了不影响已有代码,我们可以继续在扩展函数的实现上做一些扩展即可,首先我们定义一个公共的获取字典数据的方法,如下所示。
/// <summary> /// 获取字典类型的通用处理 /// </summary> /// <param name="dictTypeName">字典类型</param> /// <param name="isCache">是否缓存,默认为true</param> /// <returns></returns> private static Dictionary<string, string> GetDictByDictType(string dictTypeName, bool isCache = true) { Dictionary<string, string> dict = null; if (isCache) { System.Reflection.MethodBase method = System.Reflection.MethodBase.GetCurrentMethod(); string key = string.Format("{0}-{1}-{2}", method.DeclaringType.FullName, method.Name, dictTypeName); dict = MemoryCacheHelper.GetCacheItem<Dictionary<string, string>>(key, delegate () { return CallerFactory<IDictDataService>.Instance.GetDictByDictType(dictTypeName); }, new TimeSpan(0, 30, 0));//30分钟过期 } else { dict = CallerFactory<IDictDataService>.Instance.GetDictByDictType(dictTypeName); } return dict; }
通过使用 MemoryCacheHelper.GetCacheItem<Dictionary<string, string>> 的方式,我们可以把它设置为缓存处理方式,如果在失效时间内,则从缓存里面提取。
这样原来的绑定下拉列表的扩展方法获取字典数据,从这个公共的接口里面获取即可,而我们也仅仅是增加一个具有默认值的缓存与否的参数,用来决定是否使用缓存模式,默认为使用缓存处理。
/// <summary> /// 绑定下拉列表控件为指定的数据字典列表 /// </summary> /// <param name="control">下拉列表控件</param> /// <param name="dictTypeName">数据字典类型名称</param> /// <param name="defaultValue">控件默认值</param> /// <param name="emptyFlag">是否添加空行</param> public static void BindDictItems(this ComboBoxEdit control, string dictTypeName, string defaultValue, bool isCache = true, bool emptyFlag = true) { var dict = GetDictByDictType(dictTypeName, isCache); List<CListItem> itemList = new List<CListItem>(); foreach (string key in dict.Keys) { itemList.Add(new CListItem(key, dict[key])); } control.BindDictItems(itemList, defaultValue, emptyFlag); }
这样原来的数据下拉列表绑定的方式没有变化,依旧是我们原来的代码,但是默认采用缓存方式来绑定基于网络接口(混合框架模式)获取的字典数据。
/// <summary> /// 初始化数据字典 /// </summary> private void InitDictItem() { //初始化代码 this.txtSurgeryType.BindDictItems("手术方式"); this.txtIsFirstTime.BindDictItems("首发"); this.txtWHOGrade.BindDictItems("病理WHO分级"); this.txtLesionPart.BindDictItems("病灶部位"); this.txtOccupation.BindDictItems("病人职业"); this.txtRelapse.BindDictItems("复发"); this.txtPathologyGrade.BindDictItems("病理分级"); this.txtSymptom.BindDictItems("初发症状"); this.txtAnesthesiaMethod.BindDictItems("麻醉方法"); this.txtSpecimenDetail.BindDictItems("具体标本情况"); }
得到的编辑界面如下所示,使用缓存接口,对于大量字典数据显示的界面,界面显示速度有了不错的提升。
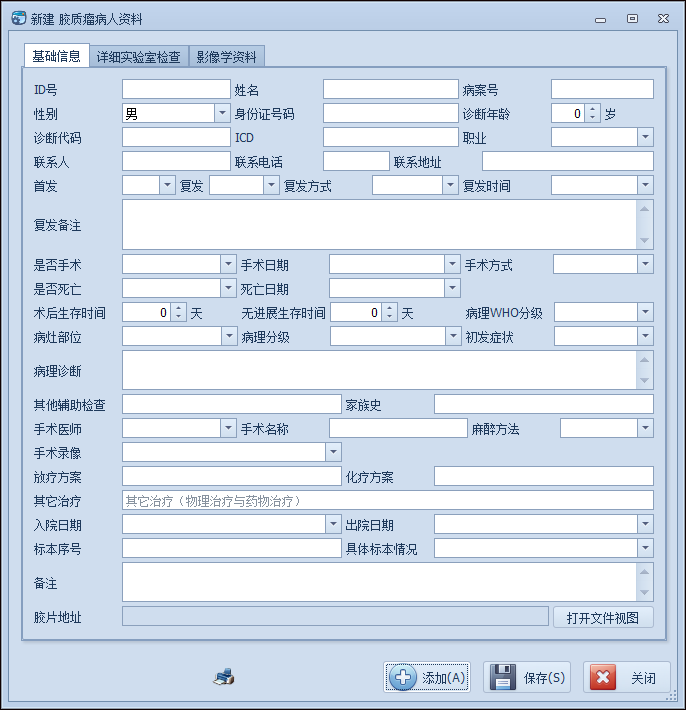
而对于一些特殊列表的字典显示,如需要通过拼音首字母进行检索功能的下拉列表,我们依旧可以使用这种绑定的方式实现缓存处理的。
如字典绑定的扩展函数如下所示,这样就统一了整个字典列表的绑定操作,比较容易记住。
/// <summary> /// 绑定下拉列表控件为指定的数据字典列表 /// </summary> /// <param name="combo">下拉列表控件</param> /// <param name="dictTypeName">数据字典类型名称</param> /// <param name="defaultValue">控件默认值</param> public static void BindDictItems(this CustomGridLookUpEdit combo, string dictTypeName, string defaultValue, bool isCache = true) { string displayName = dictTypeName; const string valueName = "值内容"; const string pinyin = "拼音码"; var dt = DataTableHelper.CreateTable(string.Format("{0},{1},{2}", displayName, valueName, pinyin)); var dict = GetDictByDictType(dictTypeName, isCache); foreach (string key in dict.Keys) { var row = dt.NewRow(); row[displayName] = key; row[valueName] = dict[key]; row[pinyin] = Pinyin.GetFirstPY(key); dt.Rows.Add(row); } combo.Properties.ValueMember = valueName; combo.Properties.DisplayMember = displayName; combo.Properties.DataSource = dt; combo.Properties.PopulateViewColumns(); combo.Properties.View.Columns[valueName].Visible = false; combo.Properties.View.Columns[displayName].Width = 400; combo.Properties.View.Columns[pinyin].Width = 200; combo.Properties.PopupFormMinSize = new System.Drawing.Size(600, 0); if (!string.IsNullOrEmpty(defaultValue)) { combo.EditValue = defaultValue; } }
界面效果如下所示。
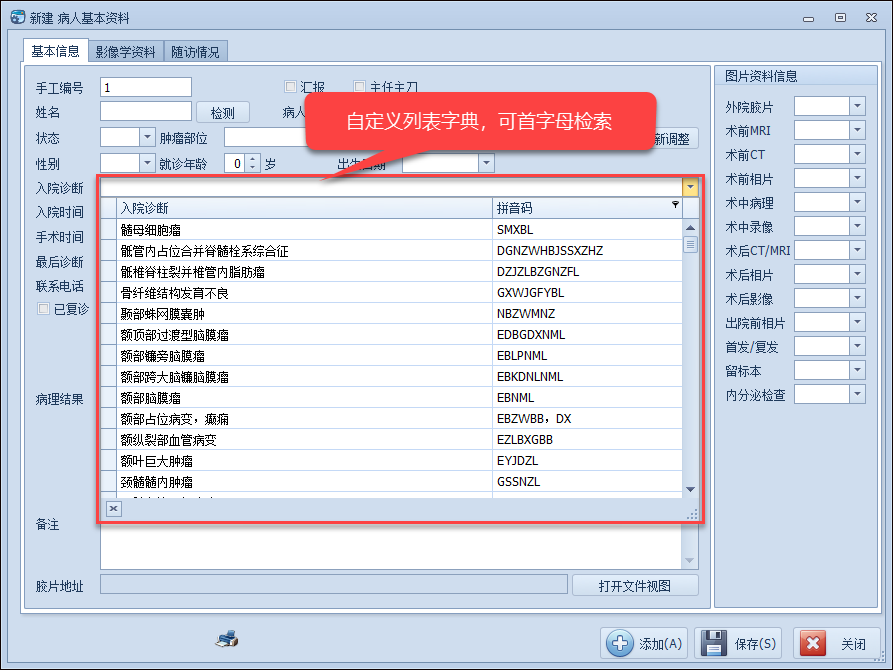
以上就是常规单机版数据绑定操作,以及基于网络版缓存数据的数据字典绑定操作,我们在界面代码的处理上没有任何差异,只是辅助扩展函数做一些调整就可以很好的变化过来了,这样对于我们界面代码的重用或者调整是非常便利的,同时缓存的使用,对于网络性能有所差异的地方,速度也会明细的有所提高。以上就是对于字典模块的一些处理上的分享,希望对大家开发Winform界面代码有所帮助和启发。
DevExpress WinForm拥有180+组件和UI库,能为Windows Forms平台创建具有影响力的业务解决方案。DevExpress WinForms能完美构建流畅、美观且易于使用的应用程序,无论是Office风格的界面,还是分析处理大批量的业务数据,它都能轻松胜任!
本文转载自:博客园 - 伍华聪
DevExpress技术交流群6:600715373 欢迎一起进群讨论
更多DevExpress线上公开课、中文教程资讯请上中文网获取

欢迎任何形式的转载,但请务必注明出处,尊重他人劳动成果
转载请注明:文章转载自:DevExpress控件中文网 [https://www.devexpresscn.com/]
本文地址:https://www.devexpresscn.com/post/3116.html